Make Laravel Modular Part 2 - Creating a Simple Caffeinated Module
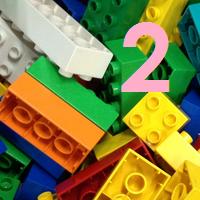
This is Part 2 of the Make Laravel Modular Series.
Tested on Laravel 5.2.*
Caffeinated Modules 3.1.*
Aimed procedure:
- Create a module placeholder
- Copy and Paste your Module files inside app/Modules
- Run the php artisan modulename--install=TRUE
- Go to http://localhost:8000/yourmodule to check
Creating a Simple Module and Controller
Let's create a Simple module.Syntax is : php artisan make:module [moduleName]
Let's do something "simple"
- php artisan make:module simple
- php artisan make:module:controller simple defaultController
- php artisan serve
- Go to http://localhost:8000/simple to check if the module is installed
"This is the Simple module index page."
The Controller
![]() |
"Instead of defining all of your request handling logic in a single routes.php file, you may wish to organize this behavior using Controller classes. Controllers can group related HTTP request handling logic into a class. Controllers are stored in the app/Http/Controllers directory." - Laravel Docs |
Let's Create a Simple Function and Route
- Open the controller in app/Module/Simple/Http/Controllers/DefaultController.php and type in something like this inside the class:
public function doSomething($var){ return view('simple::someview',['var'=>$var]) }
-
Now, doSomething() is asking for someview.blade.php
So let's create app/Modules/Simple/Resources/views/someview.blade.php and put something like this.
"The variable $var is equal to {{$var}}"
- What's with the curly brace sorrounding the $var?
- But wait, theres one more thing to do. We need to create a route, so that the user can visit our function from a browser.
Go to app/Modules/Simple/Http/routes.php and add the following lines:
Route::get('doSomething/{var}','defaultController@doSomething')
Migrate and Seed a Module
Let's say we want our Simple module to create a table with data in it.Laravel has Eloquent so we don't need to open up PHPMyAdmin or MySQL Workbench for that.
-
To create a Migration file, just run:
php artisan make:module:migration simple create_simple_table -
To create a Seeder file, just run:
php artisan make:module:seeder simple SimpleTableSeeder
Seeder file is created in app/Module/Simple/Database/Seeds/
Migrations and Seeding
![]() |
"Migrations are like version control for your database, allowing a team to easily modify and share the application's database schema. Migrations are typically paired with Laravel's schema builder to easily build your application's database schema." - Laravel Docs |
![]() |
"Laravel includes a simple method of seeding your database with test data using seed classes. All seed classes are stored in database/seeds. Seed classes may have any name you wish, but probably should follow some sensible convention, such as UsersTableSeeder, etc. By default, a DatabaseSeeder class is defined for you. From this class, you may use the call method to run other seed classes, allowing you to control the seeding order." - Laravel Docs |
Preparing a Simple Migration File
If you open app/Modules/Simple/Database/Migrations/ you will see our newly created create_simple_table.php file.Open it and we will add our table structure for the Simple table.
Inside the up() function, add this:
Schema::create('tbl_simples', function($table)
{
$table->increments('id');
$table->string('name', 50);
});
Inside the down() function, add this:
Schema::dropIfExists('tbl_simples');
It says: Create the table tbl_simples and add the columns ID which increments and is primary, and Name which is a 50 character max string.
Visit Schema Builders for more info.
Creating the Seeder File
Open app/Modules/Simple/Database/Seeds/ you will see our newly created SimpleTableSeeder.php file.Open it and we will add our table data using eloquent.
Add this just under the namespace line:
use Illuminate\Support\Facades\DB;
Inside the run() function, add this:
$data = array(
array(
'name'=>'Goku'
)
);
DB::table('tbl_simples')->insert($data);
It says: Insert row in tbl_simples with a Name value of Goku.
Visit Database Seeding for more info.
Almost Done!
We just need to call our seeder in the SimpleDatabaseSeeder.Open app/Modules/Simple/Database/Seeds/SimpleDatabaseSeeder.php.
Inside the run() function, add this:
$this->call('App\Modules\Simple\Database\Seeds\SimpleTableSeeder');
If the user seeds the module, it will call the SimpleDatabaseSeeder which will then call the SimpleTableSeeder. Visit Database Seeding for more info.
Migrate and Seed!
Now that the Migration and Seeder files are ready, let's run them.- php artisan module:migrate simple
- php artisan module:seed simple
Showing Data from tbl_simples into a Some View
Now that we have a data inside our tbl_simples, we can now call and render it in a view.To do this we need to create a model for our table. What is a model?
- php artisan make:module:model simple TblSimple
- Open app/Modules/Simple/Models/TblSimple.php
- Add this inside the class:
protected $guarded = ['id','name'];
Now the TblSimple model is binded to tbl_simples, and can manipulate data thru it. - Go back and Open your app/Modules/Simple/Http/Controllers/DefaultController.php
-
Let's import our Model in to our Controller.
Put this just below the "namespace" declaration on top.
use App\Modules\Simple\Models\TblSimple as TblSimple;
And inside the doSomething($var) function, let's call our model.
$result = TblSimple::find($var); return view('simple::someview',array('var'=>$result['name']));
It says: Import TblSimple model. And from TblSimple, find the row with the ID of $var. Return and pass the "name" field from the result to someview. - Someview.blade.php is waiting for a $var variable.
- Now access http://localhost:8000/simple/doSomething/1 and you should see Goku's name.
Comments