Make Laravel 5 Modular Part 1
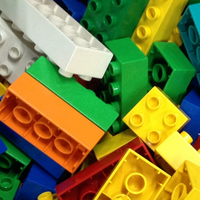
We were assigned to learn Laravel and Angular JS on our own as soon as possible.
Since I saw that the company's data is getting bigger and bigger as time goes by,
I researched for a way for us, the developers, to be able to create applications faster and make it much easier to maintain.
We all know that making a modular application is currently the best approach on large projects.
In today's business, an application must always be planned from the start to have the ability to scale as the business grows.
Some of the best advantages of the modular approach is that:
We can Reuse - Our module is created to stand alone in a Laravel 5.2 environment. This means it can live in many different Laravel instances. We can clone a module to be used by another application or another project just by injecting the Provider in to the instance.
Better maintenance - As the data in gets bigger, the requirements for its management will surely be more demanding. And with this approach, the developers will be able to isolate any issues almost instantly because all functions are segregated properly inside the modules.
Now that we have justified why we are doing this (lol), let's start the thing.
Getting Started
I will assume that you know how to use Laravel 5.2 and you have grasped the Knowledge that lies within. Well, all you need to have is a working Laravel 5.2 instance in a directory you desire and a bit of patience. But...
So I will teach you briefly how to install Laravel.
Installing Laravel 5.2
Straight to the point!
- Download and install Composer!
-
Open CMD/Shell and type this
composer global require "laravel/installer"
-
"Make sure to place the ~/.composer/vendor/bin directory
(or the equivalent directory for your OS) in
your PATHso the
laravel
executable can be located by your system."- Laravel Docs
-
Go to your Laravel projects folder then create your fresh application.
cd /path/to/your/laravel_projects
laravel new [projectName]
-
Wait for the installation to finish and still from inside the console, go inside your project directory
cd [projectName]
- You can try and SERVE your laravel instance from there by using Artisan: ( default port is 8000 but you can change it to something else.)
php artisan serve --port=8000
- http://localhost:8000/ or whatever port you used can now be accessed via internet browser.
One Laravel ala Port 8000
Now it's time to marinade Laravel.
The package behind our Modular Laravel is the Caffeinated/Modules package from Caffeinated.ninja - awesome guys, do check them out.
Installation is easy.
- Open command prompt
- Go to your New laravel directory
- Type: composer require caffeinated/modules
- Wait for the thing to finish
- Open your config/app.php
- Look for the "providers" array and add this line:
Caffeinated\Modules\ModulesServiceProvider::class, - Then look for the "aliases" array and add this line:
'Module' => Caffeinated\Modules\Facades\Module::class, - Open your config/compile.php
- Look for the "providers" array and add this line:
Caffeinated\Modules\ModulesServiceProvider::class, - Done!
Even though we can already create something awesome with this current setup, Let's go further down the road.
My aim is for the developers to not worry about the trivialities of installation from scratch and just go on and creating modules for the company's project.
So I added my own secret sauce for the scaffold, which will not be a secret anymore in a few moments.
Preparing the Environment
- Open your .env file in the root directory
- Add this line: APP_PORT=8000
- Modify the database variables to connect to your db. Make sure your DB already exists.
- Set SESSION_DRIVER=database
- To sessions will be saved in the database and needs a table.
- php artisan session:table
- php artisan migrate
- Add this line: ENV_IS_SEEN=true
Dependency Injector
Creating a module is practical because it is stand alone, and this means that dependency declaration might get redundant. Like two or more modules might require jQuery and Bootstrap, and there will be a chance that one of those will get unnecessarily redeclared. It will affect the site's response time.
It's like buying two refrigarators because your wife needs one and you also need one, then placing them side by side in the same kitchen. It's just stupid.
- Download this file: ApplicationData.php
- Then place it in your /config directory.
- Download this file: AssetScannerProvider.php
- Place it in your app/Providers directory.
- The scanner can now be injected anywhere from tha app but we'll get there in a while.
Application Data
This config file declares the default data of the application, sets the dependencies or modules to be prioritzed or ignored. It's quite self explanatory really.
Assets Scanner Provider
This provider basically asks the ApplicationData.php file what should it inject at the very top of the queue. Check the "Dependencies" array, those are the "priorities".
Preparing the Scaffold
For this to work, we need to modify the routes.php file and create a defaultController.php file.
Setting up the Controller
- Run php artisan make:controller defaultController
- This will create an app/Http/Controllers/defaultController.php file. Open it.
- Add these lines:
Just below the declarations:
use App; use App\Providers\AssetScannerProvider; and inside the class: protected $APP; public function __construct(AssetScannerProvider $assets){ $this->APP = $assets->scan(); } public function _index(){ $data = $this->APP; return view('index',$data); } public function _default(){ $data = $this->APP; return view('default',$data); } public function _navbar(){ $data = $this->APP; return view('navbar',$data); } public function _sidebar(){ $data = $this->APP; return view('sidebar',$data); } public function _footer(){ $data = $this->APP; return view('footer',$data); }
- Notice that we injected the AssetsScannerProvider on top? Yes we did...
- We also declared a protected variable $APP, and set it's value from the __construct method. __construct is basically the autoload of any controller.
- The $APP is then passed to the view.
This is how we tie the URL to the Controller.
- Open app/Http/routes.php
- Remove the default route that is declared in the file.
- Then add these lines:
Route::get('', 'defaultController@_index'); Route::get('/', 'defaultController@_index'); Route::get('default', 'defaultController@_default'); Route::get('navbar', 'defaultController@_navbar'); Route::get('sidebar', 'defaultController@_sidebar'); Route::get('footer', 'defaultController@_footer');
- It says there that if the User visits the root URL of the site, laravel will look for the method inside the defaultController and return whatever it contains.
- http://localhost:8000/navbar will call the _navbar method inside the Default Controller file.
The /public directory contains the files that are publicly available to the visitors, like Images, CSS and JS files. Asset Scanner looks for the Module directory, and so we shall create one.
- Create the public/Modules directory now. Go go go!
- Then inside it download and paste this: default.zip
- It contains some of my angular tools and SPA thingies.
If you'll notice on our Default Controller, several views are being summoned by the application. Download the Views and place them inside resources/views directory.
- Index.blade.php - Is basically the layout file, it calls the injectors, navbar, sidebar, footer directives etc... All are just modals/partials.
- You may modify the navbar.blade.php to your liking. Just make sure to escape the angular braces if you are going to use them to avoid conflict.
For example:
{{jsvariable}} should be @{{jsvariable}} -- Place an @ (at) sign in the beginning.
{{$phpVariable}} -- if you are going to call a PHP variable, just do it normally on a blade template.
Serve the Application
You may now Serve the application!
Just run php artisan serve --port=8000
APP_PORT from .env file must be the one used as --port value.
That's it for now.
Today, you learned how to install Laravel,
how to install Caffeinated Modules inside it
And how to include script and style dependencies dynamically from scratch.
LAZY DOWNLOAD:
Just paste the folders to your root directory:
Next topic, we will discuss how to create a module and we will make a module installer that we can use via php artisan.
Comments
seo melbourne